Something that has been on my mind for a long time is a interactive command interface for QGIS. Something that you can easily open, run simple commands, and is interactive to ask for arguments when they are needed.
After using the command interface in Emacs for a little bit over the weekend – you can almost hear the Boos! from heavy Vim users :) – I thought this is something I must have in QGIS as well. I’m sure it can’t be that hard to add.
So here it is. A interactive command interface for QGIS.


The command bar plugin (find it in the plugin installer) adds a simple interactive command bar to QGIS. Commands are defined as Python code and may take arguments.
Here is an example function:
@command.command("Name")
def load_project(name):
"""
Load a project from the set project paths
"""
_name = name
name += ".qgs"
for path in project_paths:
for root, dirs, files in os.walk(path):
if name in files:
path = os.path.join(root, name)
iface.addProject(path)
return
iface.addProject(_name)
All functions are interactive and if not all arguments are given when called it will prompt for each one.
Here is an example of calling the point-at
function with no args. It will ask for the x
and then the y
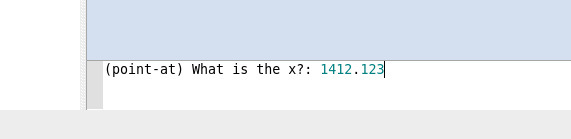
Here is calling point-at
with all the args

Functions can be called in the command bar like so:
my-function arg1 arg2 arg2
The command bar will split the line based on space and the first argument is always the function name, the rest are arguments passed to the function. You will also note that it will convert _
to -
which is easier to type and looks nicer.
The command bar also has auto complete for defined functions – and tooltips once I get that to work correctly.
You can use CTRL + ;
(CTRL + Semicolon), or CTRL + ,
, to open and close the command bar.
What is a command interface without auto complete

Use Enter to select the item in the list.
How about a function to hide all the dock panels. Sure why not.
@command.command()
def hide_docks():
docks = iface.mainWindow().findChildren(QDockWidget)
for dock in docks:
dock.setVisible(False)
alias command
You can also alias a function by calling the alias
function in the command bar.
The alias
command format is alias {name} {function} {args}
Here is an example of predefining the x for point-at
as mypoint
-> alias mypoint point-at 100
point-at
is a built in function that creates a point at x y
however we can alias it so that it will be pre-called with the x argument set. Now when we call mypoint
we only have to pass the y
each time.
-> mypoint
(point-at) What is the Y?: 200
You can even alias the alias command – because why the heck not :)
-> alias a alias
a mypoint 100
a
is now the shortcut hand for alias
WHY U NO USE PYTHON CONSOLE
The Python console is fine and dandy but we are not going for a full programming language here, that isn’t the point. The point is easy to use commands.
You could have a function called point_at
in Python that would be
point_at(123,1331)
Handling incomplete functions is a lot harder because of the Python parser. In the end it’s easier and better IMO to just make a simple DSL for this and get all the power of a DSL then try and fit into Python.
It should also be noted that the commands defined in the plugin can still be called like normal Python functions because there is no magic there. The command bar is just a DSL wrapper around them.
Notes
This is still a bit of an experiment for me so things might change or things might not work as full expected just yet.
Check out the projects readme for more info on things that need to be done, open to suggestions and pull requests.
Also see the docs page for more in depth information
Filed under:
Open Source,
python,
qgis Tagged:
plugin,
pyqgis,
qgis